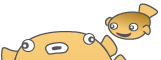
Shortening any url in Java using bitly API .
We need to get API key first using bit.ly account
In two ways we can do
1. By calling the bit.ly's REST API.
2. By using bitly core methods.
1. By calling the bit.ly's REST API
We need the following jars to work on
bitlyj-2[1].0.0
commons-httpclient-3.1
commons-codec-1.2
logging-1.0.4
Code to shorten the url
HttpClient httpclient = new HttpClient();
HttpMethod method = new GetMethod("http://api.bit.ly/shorten");
NameValuePair[] valuePair=new NameValuePair[]{new NameValuePair("longUrl","http://www.sundarishree.blogspot.com/"),
new NameValuePair("version","2.0.1"),
new NameValuePair("login","YourUsername"),
new NameValuePair("apiKey","YourApikey"),
new NameValuePair("format","xml"),
new NameValuePair("history","1")
};
method.setQueryString(valuePair);
try {
httpclient.executeMethod(method);
} catch (HttpException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
String responseXml =null;
try {
responseXml = method.getResponseBodyAsString();
} catch (IOException e) {
e.printStackTrace();
}
System.out.println(responseXml);
When the above code is excuted we will get the output in following XML format
<bitly>
<errorCode>0</errorCode>
<errorMessage></errorMessage>
<results>
<nodeKeyVal>
<shortKeywordUrl></shortKeywordUrl>
<hash>bzXiN2</hash>
<userHash>ceCaRl</userHash>
<nodeKey><![CDATA[http://www.sundarishree.blogspot.com/]]></nodeKey>
<shortUrl>http://bit.ly/ceCaRl</shortUrl>
<shortCNAMEUrl>http://bit.ly/ceCaRl</shortCNAMEUrl>
</nodeKeyVal>
</results>
<statusCode>OK</statusCode>
</bitly>
Code to get the short url from XML output
if(responseXml != null) {
// parse the XML
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
DocumentBuilder db = null;
try {
db = dbf.newDocumentBuilder();
} catch (ParserConfigurationException e) {
e.printStackTrace();
}
StringReader st = new StringReader(responseXml);
Document d = null;
String retVal=null;
try {
d = db.parse(new InputSource(st));
} catch (SAXException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
NodeList nl = d.getElementsByTagName("shortUrl");
if(nl != null) {
Node n = nl.item(0);
retVal = n.getTextContent();
System.out.println("Short URL >> "+retVal);
}
}
We can get the output as Short URL >>http://bit.ly/ceCaRl
2. By using bitly core methods
bitlyj-2[1].0.0 and commons-httpclient-3.1 jars are enough to use bitly core methods
Code to shorten the url
While importing Bitly mention as static
import static com.rosaloves.bitlyj.Bitly.*;
....
Provider bitly = Bitly.as("YourUserName", "YourApiKey");
ShortenedUrl info =bitly.call(shorten("http://www.visiontss.com/"));
System.out.println("Shorten URL "+info.getShortUrl());
output : Shorten URL http://bit.ly/ahyvK0
Bulk Methods
Some bitly methods support multiple arguments.info and clicks for example can take an arbitrary number of hash
or shortUrl arguments. You can do this in bitlyj just like you'd expect:
for(UrlInfo info1 : bitly.call(info("http://bit.ly/ceCaRl","http://bit.ly/ahyvK0"))) {
System.out.println("Created by :"
+info1.getCreatedBy());
System.out.println("ShortUrl :"
+info1.getUrl());
System.out.println("Title of the page :"
+info1.getTitle());
}
output :
Created by :sundarishree
ShortUrl :Url [shortBase=http://bit.ly/, globalHash=7haiBr, longUrl=, shortUrl=http://bit.ly/ahyvK0, userHash=ahyvK0]
Title of the page :Vision Tech Solutions - Software Product Development Company
Created by :sundarishree
ShortUrl :Url [shortBase=http://bit.ly/, globalHash=bzXiN2, longUrl=, shortUrl=http://bit.ly/ceCaRl, userHash=ceCaRl]
Title of the page :TECH.BLOG
You can know more in QuickStart
hi, i hav used bitly api in my java app to search for a key word ... can u help me debug this code...
ReplyDeletepublic class NewClass2
{
@SuppressWarnings("empty-statement")
public static void main(String[] args) throws IOException, ParserConfigurationException
{
Bitly.Provider bitly = Bitly.as("o_5vbnds3glt", "R_df8676ec0ca9da09352af6167bc34ae3");
org.apache.commons.httpclient.HttpClient httpclient = new org.apache.commons.httpclient.HttpClient();
HttpMethod method = new GetMethod("http://api.bit.ly/v3/search");
method.setQueryString(
new NameValuePair[]{
new NameValuePair("Url","http://www.sundarishree.blogspot.com/"),
// new NameValuePair("login","mylogin"),
//new NameValuePair("apiKey","mykey"),
new NameValuePair("limit","10"),
new NameValuePair("offset","0"),
new NameValuePair("query","this"),
new NameValuePair("lang","en"),
new NameValuePair("cities","us"),
new NameValuePair("domain","com"),
new NameValuePair("format","xml"),
new NameValuePair("fields","aggregate_link")
} );
// Integer s=bitly.call(
// bitly.call(httpclient.executeMethod(method));
// httpclient.executeMethod(method);
httpclient.executeMethod(method);
String responseXml = method.getResponseBodyAsString();
System.out.println(responseXml);;
}
private static void call(int executeMethod) {
throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates.
}
}
Thanks a lot really helpful, especially the bitly REST API. For those working behind a proxy server. Please use the following code
ReplyDeleteafter "HttpClient httpclient = new HttpClient();"
-----------------------------------------------------------------------------------------------------------
httpclient.getHostConfiguration().setProxy("hostname", port);
Credentials cred = new UsernamePasswordCredentials("proxyUsername","proxyPassword");
httpclient.getState().setProxyCredentials(AuthScope.ANY, cred);
There are many website allows services to shortURLs. URL-shortening services allow you to make cash when you use.
ReplyDeleteThat'sthe cause marketing that you simply appropriate research prior to creating. It's also feasible to put in writing enhanced publishing with this particular. tinyurl
ReplyDeleteWhat are some of the benefits of using URL shorteners? Perhaps the main benefit is that a link shortener is easier for copying into an email, a forum post, or a post on Twitter, where the poster is limited to 140 characters for the entire post.
ReplyDelete